일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- Sparkplug
- 주니어개발자
- task queue
- Compound Component
- type assertion
- Custom Hook
- helm-chart
- CS
- 암묵적 타입 변환
- AJIT
- Render Queue
- Redux Toolkit
- 좋은 PR
- docker
- react
- 타입 단언
- prettier-plugin-tailwindcss
- jotai
- linux 배포판
- 프로세스
- 회고
- TypeScript
- 명시적 타입 변환
- Headless 컴포넌트
- useLayoutEffect
- zustand
- 클라이언트 상태 관리 라이브러리
- JavaScript
- Recoil
- Microtask Queue
- Today
- Total
구리
TIL_210428_DAO,DTO,JSP, Servlet 활용 데이터 가공 문제 본문
목차
DAO, DTO 개념 및 설계시 주의사항
문제 1 (goodsInfo)
문제 2 (userInfo)
Q) DAO란 ?
Data Access Object로 DB 접속 및 쿼리문 처리 담당하는 역할을 한다
Q) DAO / DTO 차이는 ?
DAO는 DB에 접근하기 위한 객체고 DTO는 데이터 교환을 위해 임의로 만든 객체라고 생각하면 된다
(DAO가 데이터를 가지고 있는 게 아니다)
DTO 설계시 주의사항
* 멤버변수명 설정
1. <input type ~~ name="파라미터명">
2. DTO 클래스 멤버변수명
3. DB 테이블 필드명
위 세개가 다 동일한 이름으로 설정한다.
* 멤버변수 접근 제한자
모든 멤버변수는 private으로 접근제한자를 설정하며 변수에 접근하기 위한 gettet/setter 메소드 필수 선언
* 기본 생성자 필수 선언
자바 기본 작업시에는main()을 이용하기에 매개변수 생성자가 없다면 클래스 컴파일시 기본 생성자가 자동생성되었지만
자바 웹 작업에서는 톰캣이 모든 일을 주관하기에 추후 injection 개념에서 오류 발생
DAO 설계시 주의 사항
* 외부에서 객체 생성 못하도록 기본 생성자에 private 접근 제한자 명시
* 내부에서 객체 반환 할 수 있도록 public 메서드 생성
* Connection 객체는 단 한개만 생성될 수 있도록 작업
(클래스 static 멤버 변수로 선언, 이유 ? DB 접속은 1번만 이뤄지면 되기에 -> SingleTone 패턴)
문제 1) 상품을 조회하고 수정할 수 있는 기능을 갖춘 프로젝트를 만드시오
(단, 사용자 화면은 jsp 페이지에서, 데이터 관련 과정들은 서블릿, 자바 파일을 사용하시오)
(사진에 나온 GoodsInfoReader.jsp, GoodsInfoUpdate.jsp는 제외)
각 파일의 역할
GoodsInfoInitForm.jsp - 코드 검색하는 화면
GoodsInfoViewer.jsp - 검색 조건에 따른 결과 화면
GoodsInfoEditForm.jsp - 검색한 데이터를 수정하는 화면
SearchServlet.java - 조회 요청이 들어오면 GoodsDAO 클래스 메서드 이용해 원하는 데이터를 반환하는 서블릿
UpdateServlet.java - 데이터 수정 요청이 들어오면 GoodsDAO 클래스 메서드 이용해 원하는 데이터를 반환하는 서블릿
GoodsDAO.java - 서블릿에서 요청이 들어오면 데이터를 불러와 실제 요청 처리를 하는 클래스
GoodsDTO.java - 원하는 데이터를 저장하여 옮기기 위한 수단을 담당하는 클래스
goodsinfo 테이블 구조
goodsinfo 테이블 데이터
문제 설계도
프로젝트 코드
GoodsInfoInitForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" errorPage="DBError.jsp"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>상품 정보 조회</title>
</head>
<body>
<h3>상품코드를 입력하세요</h3>
<form action="search">
상품코드:
<input type="text" name="code" size="5" maxlength="5" required="required" /><br />
<input type="submit" value="확인" />
</form>
</body>
</html>
SearchServlet.java
package com.bjy.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import com.bjy.dao.GoodsDAO;
import com.bjy.dto.GoodsDTO;
@WebServlet("/search")
public class SearchServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("SerachServlet의 doGet() 실행"); // 이클립스 콘솔 확인
// 1. GoodsInfoInitForm.jsp로부터 전달된 파라터값 추출
String code = request.getParameter("code");
// 2.GoodsDAO 객체 생성
GoodsDAO dao = GoodsDAO.getInstance();
// 3. GoodsDAO 객체의 getGoods() 메서드 호출 후 결과값 받기
GoodsDTO dto = dao.getGoods(code);
// forward() 하기 전 테스트
// dto.goodsToString();
// 4. 결과값을 GoodsInfoViewer.jsp 에게 전달
HttpSession session = request.getSession(); // 서블릿 내에서 세션 객체 얻기
session.setAttribute("DTO", dto);
response.sendRedirect("GoodsInfoViewer.jsp");
// 데이터를 세션에 저장했으므로 굳이 forward() 쓸 이유 없음
// RequestDispatcher rd = request.getRequestDispatcher("GoodsInfoViewer.jsp");
// rd.forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
GoodsDAO.java
package com.bjy.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.bjy.dto.GoodsDTO;
import jdk.nashorn.internal.ir.RuntimeNode.Request;
/** 데이터베이스 접속, 쿼리 실행에 관련된 모든 메서드 보유 : 디자인 패턴은 SingleTone Pattern **/
public class GoodsDAO {
public static GoodsDAO instance = null;
private GoodsDAO() {
}
public static GoodsDAO getInstance() {
if(instance == null) {
instance = new GoodsDAO();
}
return instance;
}
public Connection getConnection() {
Connection conn = null;
String url = "jdbc:oracle:thin:@127.0.0.1:1521:XE";
String userid = "bjy";
String userpw = "qorwjddus96";
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
conn = DriverManager.getConnection(url, userid, userpw);
} catch (ClassNotFoundException e) {
System.out.println("GoodsDAO- 드라이버 로드 ERR : " + e.getMessage());
e.printStackTrace();
} catch (SQLException e) {
System.out.println("GoodsDAO - 커넥트 ERR : " + e.getMessage());
}
return conn;
} // end of getConnection()
/** 코드를 이용하여 해당 데이터를 추출하여 반환 **/
/** getGoods()는 SearchServlet가 호출**/
/** 결과인 GoodsDTO dto를 SearchServlet에게 반환 **/
public GoodsDTO getGoods(String code) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String query = "select * from goodsinfo where code=?";
GoodsDTO dto = null;
try {
conn = getConnection();
pstmt = conn.prepareStatement(query);
pstmt.setString(1, code);
rs = pstmt.executeQuery();
if(rs.next()) {
dto = new GoodsDTO();
dto.setCode(rs.getString("code"));
dto.setTitle(rs.getString("title"));
dto.setWriter(rs.getString("writer"));
dto.setPrice(rs.getInt("price"));
}
}catch(SQLException e) {
System.out.println();
}finally{
try {
// 데이터를 dto 객체에 저장했기 때문에 rs 객체 필요없어짐
if(rs != null) {
rs.close();
}
if(pstmt != null) {
pstmt.close();
}
if(conn != null) {
conn.close();
}
}catch(SQLException e) {
System.out.println("GoodsDAO - CLOSE ERR : " + e.getMessage());
}
} // end of finally
return dto;
} // end of getGoods(String code)
/** 코드를 이용하여 해당 레코드의 데이터를 수정 **/
public void updateGoods(GoodsDTO dto) {
Connection conn = null;
PreparedStatement pstmt = null;
String query = "update goodsinfo set title=?, writer=?, price=? where code=?";
try {
conn = getConnection();
pstmt = conn.prepareStatement(query);
pstmt.setString(1, dto.getTitle());
pstmt.setString(2, dto.getWriter());
pstmt.setInt(3, dto.getPrice());
pstmt.setString(4, dto.getCode());
int n = pstmt.executeUpdate();
if(n>0) {
System.out.println("GoodsDAO.java -updateGoods() : 데이터 수정 완료 ^^ ");
}else {
System.out.println("GoodsDAO.java -updateGoods() : 데이터 수정 실패 ! ");
}
}catch(SQLException e) {
System.out.println();
}finally{
try {
if(pstmt != null) {
pstmt.close();
}
if(conn != null) {
conn.close();
}
}catch(SQLException e) {
System.out.println("GoodsDAO - CLOSE ERR : " + e.getMessage());
}
} // end of finally
}
}
GoodsDTO.java
package com.bjy.dto;
/** 요청, 결과 데이터를 싣고 다니는 역할 **/
public class GoodsDTO {
// 멤버변수 초기화 필수 !!!
// db 테이블 필드명, input 태그 파라미터명과 동일하게 설정
private String code = null;
private String title = null;
private String writer = null;
private int price = 0;
// 기본 생성자 필수
public GoodsDTO() {
}
// 멤버변수 다루기 위한 getter/setter 메서드 선언
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getWriter() {
return writer;
}
public void setWriter(String writer) {
this.writer = writer;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public void goodsToString() {
System.out.println(this.code + "," + this.title + "," + this.writer + "," + this.price);
}
}
GoodsInfoViewer.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="com.bjy.dto.GoodsDTO" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>상품 정보 출력</title>
</head>
<body>
<h3>상품 정보</h3>
코드 : ${DTO.getCode() }<br />
제목 : ${DTO.getTitle() }<br />
저자 : ${DTO.getWriter() }<br />
가격 : <fmt:formatNumber value="${DTO.getPrice() }" type="currency"></fmt:formatNumber><br />
<input type="button" value="수정" onclick="document.location.href='GoodsInfoEditForm.jsp'"/>
</body>
</html>
GoodsInfoInitForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>상품 정보 수정</title>
</head>
<body>
<h4>상품 정보를 수정한 후 수정 버튼을 누르십시오.</h4>
<form action="update" method="post">
코드: <input type="text" name="code" size="5" value="${DTO.getCode() }" readonly="readonly" /> <br />
제목: <input type="text" name="title" size="50" placeholder="${DTO.getTitle() }"/> <br />
저자: <input type="text" name="writer" size="20" placeholder="${DTO.getWriter()}"/> <br />
가격: <input type="text" name="price" size="8" placeholder="${DTO.getPrice() }"/>원 <br />
<input type="submit" value="수정" />
</form>
</body>
</html>
UpdateServlet.java
package com.bjy.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.bjy.dao.GoodsDAO;
import com.bjy.dto.GoodsDTO;
@WebServlet("/update")
public class UpdateServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("GoodsInfoEditForm.jsp에서 doGet()메서드 호출 ");
// 1. GoodsInfoEditForm.jsp에서 수정된 값 추출
String code = request.getParameter("code");
String title = request.getParameter("title");
String writer = request.getParameter("writer");
int price = Integer.parseInt(request.getParameter("price"));
// 2. GoodsDAO 객체 생성
GoodsDAO dao = GoodsDAO.getInstance();
// 3. GoodsDAO 객체의 updateGoods()에게 추출된 값 전달
// 1) 파리미터값 4개를 매개변수로 보냄 / 2) 파라미터값을 dto 객체 생성 후 멤버변수로 설정하여 dto 객체 1개를 매개변수로 보냄
GoodsDTO dto = new GoodsDTO();
dto.setCode(code);
dto.setTitle(title);
dto.setWriter(writer);
dto.setPrice(price);
dao.updateGoods(dto);
// 4. 결과값 확인을 위해 GoodsInfoInitForm.jsp 로 이동
response.sendRedirect("GoodsInfoInitForm.jsp");
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// GoodsInfoEditForm.jsp form 태그가 post 형식일 경우 문자열 인코딩 설정만 해주면 끝 !
// doGet() 코드 복붙할 필요 X
request.setCharacterEncoding("UTF-8");
doGet(request, response);
}
}
Q) SearchServlet 서블릿에서 세션은 그냥 사용해도 되지 않나 ?
JSP에선 session이 내장 객체기에 별도의 객체 생성 메소드 없이 사용 가능했지만
Servlet에선 session 객체 생성 메소드인 request.getSession() 필수 호출!
결과 사진




문제2) DB 테이블 userinfo를 이용하여 문제 1과 같은 흐름을 지니는 프로젝트를 생성하시오 (사용자 가입할 수 있는 기능 및 화면 추가)
(단 userinfo 테이블 데이터는 임의로 설정한다)
각 파일 역할
UserInfoInitForm.jsp - 사용자 이름으로 검색하는 화면
UserInfoViewer.jsp - 검색 조건에 따른 결과 화면
UserInfoEditForm.jsp - 검색한 사용자 정보를 수정하는 화면
UserInfoJoinForm.jsp - 가입에 필요한 정보를 작성하는 화면
SearchServlet.java - 사용자 조회 요청이 들어오면 GoodsDAO 클래스 메서드 이용해 원하는 데이터를 반환하는 서블릿
UpdateServlet.java - 데이터 수정 요청이 들어오면 GoodsDAO 클래스 메서드 이용해 원하는 데이터를 반환하는 서블릿
JoinServlet.java - 사용자 가입 요청이 들어오면 GoodsDAO 클래스 메서드 이용해 원하는 데이터를 반환하는 서블릿
UserDAO.java - 서블릿에서 요청이 들어오면 데이터를 불러와 실제 요청 처리를 하는 클래스
UserDTO.java - 원하는 데이터를 저장하여 옮기기 위한 수단을 담당하는 클래스
userinfo 테이블 구조
userinfo 테이블 데이터
문제 설계도
직접 짠 코드
UserInfoInitForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>User 조회 화면</title>
</head>
<body>
<form action="search">
유저 이름 조회 :
<input type="text" name="name" required="required" size="50" maxlength="50" /><br />
<input type="submit" value="조회" />
<input type="button" value="가입" onclick="location.href='UserInfoJoinForm.jsp'">
</form>
</body>
</html>
SearchServlet.java
package com.bjy.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import com.bjy.dao.UserDAO;
import com.bjy.dto.UserDTO;
@WebServlet("/search")
public class SearchServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 테스트용
// System.out.println("searchservlet의 doGet() 실행~");
String name = request.getParameter("name");
UserDAO dao = UserDAO.getInstance();
UserDTO dto = new UserDTO();
dto = dao.searchUser(name);
// 테스트용
// dto.dtoToString();
HttpSession session = request.getSession();
session.setAttribute("DTO", dto);
response.sendRedirect("UserInfoViewer.jsp");
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
UserDAO.java
package com.bjy.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.bjy.dto.UserDTO;
public class UserDAO {
private static UserDAO instance = null;
public UserDAO() {
}
public static UserDAO getInstance() {
if(instance == null) {
instance = new UserDAO();
}
return instance;
}
public Connection connectDB() {
String url = "jdbc:oracle:thin:@127.0.0.1:1521:XE";
String userid = "bjy";
String userpw = "qorwjddus96";
Connection conn = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
conn = DriverManager.getConnection(url, userid, userpw);
}catch(ClassNotFoundException e) {
System.out.println("UserDAO.java - connectDB() : driver ERR : " + e.getMessage());
} catch (SQLException e) {
System.out.println("UserDAO.java - connectDB() : connection ERR : " + e.getMessage());
}
return conn;
}
public UserDTO searchUser(String name) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String query = "select * from userinfo where name=?";
UserDTO dto = null;
try {
conn = connectDB();
pstmt = conn.prepareStatement(query);
pstmt.setString(1, name);
rs = pstmt.executeQuery();
rs.next();
dto = new UserDTO();
dto.setName(rs.getString("name"));
dto.setId(rs.getString("id"));
dto.setPassword(rs.getString("password"));
}catch(SQLException e) {
System.out.println("UserDAO.java - searchUser() ERR : " + e.getMessage());
}finally {
try {
if(rs != null) {
rs.close();
}
if(pstmt != null) {
pstmt.close();
}
if(conn != null) {
conn.close();
}
}catch(SQLException e) {
System.out.println("UserDAO.java - searchUser() : close() ERR : " + e.getMessage());
}
}
return dto;
}
public void updateUser(String id, String password) {
Connection conn = null;
PreparedStatement pstmt = null;
String query = "update userinfo set password=? where id=?";
try {
conn = connectDB();
pstmt = conn.prepareStatement(query);
pstmt.setString(1, password);
pstmt.setString(2, id);
int n = pstmt.executeUpdate();
if(n>0) {
System.out.println("데이터 수정 완료 !");
}else {
System.out.println("데이터 수정 실패...");
}
}catch(SQLException e) {
System.out.println("UserDAO.java - updateUser() ERR : " + e.getMessage());
}finally {
try {
if(pstmt != null) {
pstmt.close();
}
if(conn != null) {
conn.close();
}
}catch(SQLException e) {
System.out.println("UserDAO.java - updateUser() : close() ERR : " + e.getMessage());
}
}
} // end of updateUser()
public void joinUser(UserDTO dto) {
Connection conn = null;
PreparedStatement pstmt = null;
String query = "insert into userinfo values (?,?,?)";
try {
conn = connectDB();
pstmt = conn.prepareStatement(query);
pstmt.setString(1, dto.getName());
pstmt.setString(2, dto.getId());
pstmt.setString(3, dto.getPassword());
int n =pstmt.executeUpdate();
if(n>0) {
System.out.println("DB 데이터 삽입 성공 !");
}else {
System.out.println("DB 데이터 삽입 실패...");
}
}catch(SQLException e) {
System.out.println("UserDAO - joinUser() ERR : " + e.getMessage());
}finally {
try {
if(pstmt != null) {
pstmt.close();
}
if(conn != null) {
conn.close();
}
}catch(SQLException e) {
System.out.println("UserDAO - joinUser() close() ERR : " + e.getMessage() );
}
}
}
}
UserDTO.java
package com.bjy.dto;
public class UserDTO {
private String name = null;
private String id = null;
private String password = null;
public UserDTO() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public void dtoToString() {
System.out.println(this.name + "," + this.id + "," + this.password);
}
}
UserInfoViewer.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>조회된 유저 정보 출력</title>
</head>
<body>
<h3>조회된 유저 정보</h3>
<form action="update"></form>
<table border="1">
<tr>
<td>이름</td>
<td>아이디</td>
<td>비밀번호</td>
</tr>
<tr>
<td>${DTO.getName() }</td>
<td>${DTO.getId() }</td>
<td>${DTO.getPassword() }</td>
</tr>
</table>
<input type="button" value="수정" onclick="location.href='UserInfoEditForm.jsp'">
</body>
</html>
UserInfoEditForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>유저 정보 수정 화면</title>
</head>
<body>
<h3>유저 정보를 수정하세요</h3>
<form action="update" method="post">
이름:<input type="text" name="name" value="${DTO.getName() }" readonly="readonly" /><br />
id:<input type="text" name="id" value="${DTO.getId() }" readonly="readonly" /><br />
password:<input type="text" name="password" value="${DTO.getPassword() }" /><br />
<input type="submit" value="저장" />
</form>
</body>
</html>
UpdateServlet.jsp
package com.bjy.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.bjy.dao.UserDAO;
import com.bjy.dto.UserDTO;
@WebServlet("/update")
public class UpdateServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String id = request.getParameter("id");
String password = request.getParameter("password");
UserDAO dao = UserDAO.getInstance();
dao.updateUser(id, password);
response.sendRedirect("UserInfoInitForm.jsp");
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
doGet(request, response);
}
}
UserInfoJoinForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>유저 가입 화면</title>
</head>
<body>
<h3>가입을 위한 정보를 모두 입력해주세요.</h3>
<form action="join" method="post">
이름 : <input type="text" name="name" size="20" required="required" /><br />
아이디 : <input type="text" name="id" size="20" required="required" /><br />
비밀번호 : <input type="password" name="password" size="20" required="required" /><br />
<input type="submit" value="전송" />
</form>
</body>
</html>
결과사진




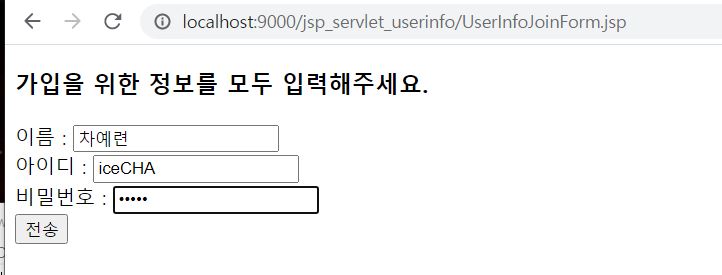

'JSP,Serlvet' 카테고리의 다른 글
TIL_210531_model1,model2,MVC 패턴 (0) | 2021.05.31 |
---|---|
TIL_210429_JSP,Servlet 활용 로그인 및 회원가입 프로그램 (2) | 2021.04.29 |
210427_JSP 관련 데이터 다루기 문제 (0) | 2021.04.27 |
TIL_210423_쿠키, 세션 (0) | 2021.04.27 |
TIL_210426_JSTL ,Servlet 기초 (0) | 2021.04.26 |